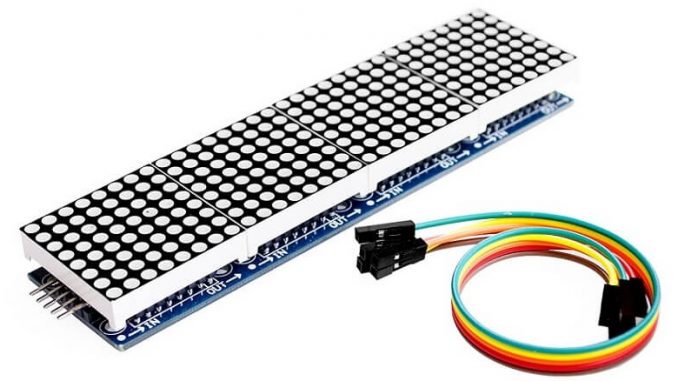
Pada artikel berikut ini akan dijelaskan mengenai modul Display Dot Matrix MAX7219 4 In 1 yang banyak tersedia di pasaran, beserta tutorial koneksi rangkaian dengan Arduio serta contoh program nya.
Pengertian
MAX7219 Dot Matrix 4 In 1 Display Module adalah sebuah papan display yang disusun secara seri dari 4 buah 8 x 8 Dot Matrix dengan menggunakan IC kontroller MAX7219. Pada dasarnya MAX7219 merupakan sebuah IC Shift Register yang khusus dirancang untuk mengontrol Dot Matrix, 7 Segment maupun independen LED.
1 buah IC MAX7219 bisa digunakan untuk mengontrol :
- 1 buah Dot Matrix 8 x 8
- 8 buah 7 Segment
- 64 independen LED
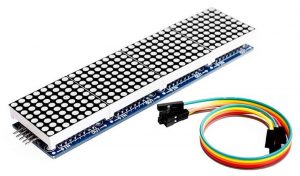
Oleh karena menggunakan mekanisme Shift Register tersebut maka IC MAX7219 hanya menggunakan 3 pin input untuk mengontrol ketiga display diatas, yaitu : D IN, CS dan CLK disamping tentunya VCC dan GND.
Untuk mempelajari lebih detail terkait pin out dan cara kerja dari IC MAX7219, sehingga anda bisa membuat atau design sendiri atau custom display Dot Matrix maupun 7 Segment sesuai kebutuhan atau project, silahkan buka link artikel dibawah :
Pelajari Lebih detail : IC MAX7219 – 8 Digit 7 Segment, Dot Matrix 8×8 LED Display Driver IC
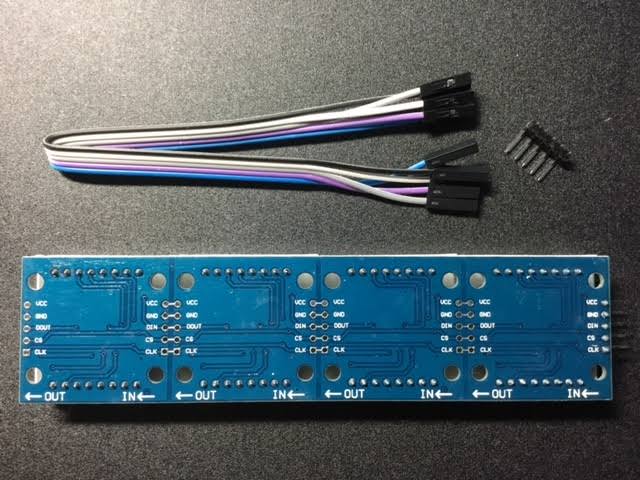
Jika kita ingin dapat mengontrol lebih banyak display, maka tinggal disusun secara seri sesuai jumlah display yang diinginkan, seperti terlihat pada gambar diatas.
Spesifikasi
Berikut adalah spesifikasi dari modul display Dot Matrix MAX7219 4 In 1 :
- Tegangan Operasi : 5V
- Terdiri dari 4 buah Dot Matrix 8 x 8 yang tersusun secara seri.
- Menggunakan IC kontroller MAX7219
- Dimensi / ukuran : 12.8 cm x 12.8 cm x 1.3 cm
Hardware Yang Dibutuhkan
Hardware yang diperlukan pada contoh program modul Dot Matrix MAX7219 4 In 1 dengan Arduino pada tutorial ini antara lain :
- Modul Dot Matrix MAX7219 4 In 1
- Arduino UNO / Arduino Nano / Arduino Pro Mini atau lainnya
- Beberapa kabel jumper M-F / F-F
Rangkaian / Wiring Diagram
Berikut ini merupakan rangkaian atau sambungan antara Arduino dengan MAX7219 Display Module.
MAX7219 Dot Matrix Module | Arduino Nano / Pro Mini / Lainnya |
VCC | 5V |
GND | GND |
D IN | D12 |
CLK | D11 |
CS | D10 |
Kode Program
Keuntungan menggunakan Arduino adalah kemudahan dalam penggunaan dan juga dukungan pengembangan dari komunitas yang luas. Karenanya untuk menggunakan MAX7219 Dot Matrix Modul ini, kita tidak perlu repot lagi sebab sudah tersedia library yang dikembangkan oleh komunitas pengguna Arduino yang bisa didownload pada link berikut :
Setelah library LedControl.zip diatas didownload, kemudian ekstrak dan copy ke folder library dari Arduino IDE. Jika Arduino IDE dalam kondisi terbuka, maka tutup terlebih dahulu trus buka kembali.
Berikut ini adalah contoh sketch untuk menyalakan satu per satu dari LED Dot Matrix kemudian mematikannya satu per satu dan dilakukan secara berulang terus menerus.
#include "LedControl.h" // need the library LedControl lc=LedControl(12,11,10,1); // // pin 12 is connected to the MAX7219 pin 1 // pin 11 is connected to the CLK pin 13 // pin 10 is connected to LOAD pin 12 // 1 as we are only using 1 MAX7219 void setup() { // the zero refers to the MAX7219 number, it is zero for 1 chip lc.shutdown(0,false);// turn off power saving, enables display lc.setIntensity(0,8);// sets brightness (0~15 possible values) lc.clearDisplay(0);// clear screen } void loop() { for (int row=0; row<8; row++) { for (int col=0; col<8; col++) { lc.setLed(0,col,row,true); // turns on LED at col, row delay(25); } } for (int row=0; row<8; row++) { for (int col=0; col<8; col++) { lc.setLed(0,col,row,false); // turns off LED at col, row delay(25); } } }
Sedangkan berikut ini adalah contoh program untuk menuliskan text / kalimat pada LED Dot Matrix kemudian tulisan tersebut discroll.
#include <avr/pgmspace.h> #include <LedControl.h> const int numDevices = 2; // number of MAX7219s used const long scrollDelay = 75; // adjust scrolling speed unsigned long bufferLong [14] = {0}; LedControl lc=LedControl(12,11,10,numDevices); prog_uchar scrollText[] PROGMEM ={ " THE QUICK BROWN FOX JUMPED OVER THE LAZY DOG 1234567890 the quick brown fox jumped over the lazy dog \0"}; void setup(){ for (int x=0; x<numDevices; x++){ lc.shutdown(x,false); //The MAX72XX is in power-saving mode on startup lc.setIntensity(x,8); // Set the brightness to default value lc.clearDisplay(x); // and clear the display } } void loop(){ scrollMessage(scrollText); scrollFont(); } /////////////////////////////////////////////////////////////////////////////////////////////////////////////////// prog_uchar font5x7 [] PROGMEM = { //Numeric Font Matrix (Arranged as 7x font data + 1x kerning data) B00000000, //Space (Char 0x20) B00000000, B00000000, B00000000, B00000000, B00000000, B00000000, 6, B10000000, //! B10000000, B10000000, B10000000, B00000000, B00000000, B10000000, 2, B10100000, //" B10100000, B10100000, B00000000, B00000000, B00000000, B00000000, 4, B01010000, //# B01010000, B11111000, B01010000, B11111000, B01010000, B01010000, 6, B00100000, //$ B01111000, B10100000, B01110000, B00101000, B11110000, B00100000, 6, B11000000, //% B11001000, B00010000, B00100000, B01000000, B10011000, B00011000, 6, B01100000, //& B10010000, B10100000, B01000000, B10101000, B10010000, B01101000, 6, B11000000, //' B01000000, B10000000, B00000000, B00000000, B00000000, B00000000, 3, B00100000, //( B01000000, B10000000, B10000000, B10000000, B01000000, B00100000, 4, B10000000, //) B01000000, B00100000, B00100000, B00100000, B01000000, B10000000, 4, B00000000, //* B00100000, B10101000, B01110000, B10101000, B00100000, B00000000, 6, B00000000, //+ B00100000, B00100000, B11111000, B00100000, B00100000, B00000000, 6, B00000000, //, B00000000, B00000000, B00000000, B11000000, B01000000, B10000000, 3, B00000000, //- B00000000, B11111000, B00000000, B00000000, B00000000, B00000000, 6, B00000000, //. B00000000, B00000000, B00000000, B00000000, B11000000, B11000000, 3, B00000000, /// B00001000, B00010000, B00100000, B01000000, B10000000, B00000000, 6, B01110000, //0 B10001000, B10011000, B10101000, B11001000, B10001000, B01110000, 6, B01000000, //1 B11000000, B01000000, B01000000, B01000000, B01000000, B11100000, 4, B01110000, //2 B10001000, B00001000, B00010000, B00100000, B01000000, B11111000, 6, B11111000, //3 B00010000, B00100000, B00010000, B00001000, B10001000, B01110000, 6, B00010000, //4 B00110000, B01010000, B10010000, B11111000, B00010000, B00010000, 6, B11111000, //5 B10000000, B11110000, B00001000, B00001000, B10001000, B01110000, 6, B00110000, //6 B01000000, B10000000, B11110000, B10001000, B10001000, B01110000, 6, B11111000, //7 B10001000, B00001000, B00010000, B00100000, B00100000, B00100000, 6, B01110000, //8 B10001000, B10001000, B01110000, B10001000, B10001000, B01110000, 6, B01110000, //9 B10001000, B10001000, B01111000, B00001000, B00010000, B01100000, 6, B00000000, //: B11000000, B11000000, B00000000, B11000000, B11000000, B00000000, 3, B00000000, //; B11000000, B11000000, B00000000, B11000000, B01000000, B10000000, 3, B00010000, //< B00100000, B01000000, B10000000, B01000000, B00100000, B00010000, 5, B00000000, //= B00000000, B11111000, B00000000, B11111000, B00000000, B00000000, 6, B10000000, //> B01000000, B00100000, B00010000, B00100000, B01000000, B10000000, 5, B01110000, //? B10001000, B00001000, B00010000, B00100000, B00000000, B00100000, 6, B01110000, //@ B10001000, B00001000, B01101000, B10101000, B10101000, B01110000, 6, B01110000, //A B10001000, B10001000, B10001000, B11111000, B10001000, B10001000, 6, B11110000, //B B10001000, B10001000, B11110000, B10001000, B10001000, B11110000, 6, B01110000, //C B10001000, B10000000, B10000000, B10000000, B10001000, B01110000, 6, B11100000, //D B10010000, B10001000, B10001000, B10001000, B10010000, B11100000, 6, B11111000, //E B10000000, B10000000, B11110000, B10000000, B10000000, B11111000, 6, B11111000, //F B10000000, B10000000, B11110000, B10000000, B10000000, B10000000, 6, B01110000, //G B10001000, B10000000, B10111000, B10001000, B10001000, B01111000, 6, B10001000, //H B10001000, B10001000, B11111000, B10001000, B10001000, B10001000, 6, B11100000, //I B01000000, B01000000, B01000000, B01000000, B01000000, B11100000, 4, B00111000, //J B00010000, B00010000, B00010000, B00010000, B10010000, B01100000, 6, B10001000, //K B10010000, B10100000, B11000000, B10100000, B10010000, B10001000, 6, B10000000, //L B10000000, B10000000, B10000000, B10000000, B10000000, B11111000, 6, B10001000, //M B11011000, B10101000, B10101000, B10001000, B10001000, B10001000, 6, B10001000, //N B10001000, B11001000, B10101000, B10011000, B10001000, B10001000, 6, B01110000, //O B10001000, B10001000, B10001000, B10001000, B10001000, B01110000, 6, B11110000, //P B10001000, B10001000, B11110000, B10000000, B10000000, B10000000, 6, B01110000, //Q B10001000, B10001000, B10001000, B10101000, B10010000, B01101000, 6, B11110000, //R B10001000, B10001000, B11110000, B10100000, B10010000, B10001000, 6, B01111000, //S B10000000, B10000000, B01110000, B00001000, B00001000, B11110000, 6, B11111000, //T B00100000, B00100000, B00100000, B00100000, B00100000, B00100000, 6, B10001000, //U B10001000, B10001000, B10001000, B10001000, B10001000, B01110000, 6, B10001000, //V B10001000, B10001000, B10001000, B10001000, B01010000, B00100000, 6, B10001000, //W B10001000, B10001000, B10101000, B10101000, B10101000, B01010000, 6, B10001000, //X B10001000, B01010000, B00100000, B01010000, B10001000, B10001000, 6, B10001000, //Y B10001000, B10001000, B01010000, B00100000, B00100000, B00100000, 6, B11111000, //Z B00001000, B00010000, B00100000, B01000000, B10000000, B11111000, 6, B11100000, //[ B10000000, B10000000, B10000000, B10000000, B10000000, B11100000, 4, B00000000, //(Backward Slash) B10000000, B01000000, B00100000, B00010000, B00001000, B00000000, 6, B11100000, //] B00100000, B00100000, B00100000, B00100000, B00100000, B11100000, 4, B00100000, //^ B01010000, B10001000, B00000000, B00000000, B00000000, B00000000, 6, B00000000, //_ B00000000, B00000000, B00000000, B00000000, B00000000, B11111000, 6, B10000000, //` B01000000, B00100000, B00000000, B00000000, B00000000, B00000000, 4, B00000000, //a B00000000, B01110000, B00001000, B01111000, B10001000, B01111000, 6, B10000000, //b B10000000, B10110000, B11001000, B10001000, B10001000, B11110000, 6, B00000000, //c B00000000, B01110000, B10001000, B10000000, B10001000, B01110000, 6, B00001000, //d B00001000, B01101000, B10011000, B10001000, B10001000, B01111000, 6, B00000000, //e B00000000, B01110000, B10001000, B11111000, B10000000, B01110000, 6, B00110000, //f B01001000, B01000000, B11100000, B01000000, B01000000, B01000000, 6, B00000000, //g B01111000, B10001000, B10001000, B01111000, B00001000, B01110000, 6, B10000000, //h B10000000, B10110000, B11001000, B10001000, B10001000, B10001000, 6, B01000000, //i B00000000, B11000000, B01000000, B01000000, B01000000, B11100000, 4, B00010000, //j B00000000, B00110000, B00010000, B00010000, B10010000, B01100000, 5, B10000000, //k B10000000, B10010000, B10100000, B11000000, B10100000, B10010000, 5, B11000000, //l B01000000, B01000000, B01000000, B01000000, B01000000, B11100000, 4, B00000000, //m B00000000, B11010000, B10101000, B10101000, B10001000, B10001000, 6, B00000000, //n B00000000, B10110000, B11001000, B10001000, B10001000, B10001000, 6, B00000000, //o B00000000, B01110000, B10001000, B10001000, B10001000, B01110000, 6, B00000000, //p B00000000, B11110000, B10001000, B11110000, B10000000, B10000000, 6, B00000000, //q B00000000, B01101000, B10011000, B01111000, B00001000, B00001000, 6, B00000000, //r B00000000, B10110000, B11001000, B10000000, B10000000, B10000000, 6, B00000000, //s B00000000, B01110000, B10000000, B01110000, B00001000, B11110000, 6, B01000000, //t B01000000, B11100000, B01000000, B01000000, B01001000, B00110000, 6, B00000000, //u B00000000, B10001000, B10001000, B10001000, B10011000, B01101000, 6, B00000000, //v B00000000, B10001000, B10001000, B10001000, B01010000, B00100000, 6, B00000000, //w B00000000, B10001000, B10101000, B10101000, B10101000, B01010000, 6, B00000000, //x B00000000, B10001000, B01010000, B00100000, B01010000, B10001000, 6, B00000000, //y B00000000, B10001000, B10001000, B01111000, B00001000, B01110000, 6, B00000000, //z B00000000, B11111000, B00010000, B00100000, B01000000, B11111000, 6, B00100000, //{ B01000000, B01000000, B10000000, B01000000, B01000000, B00100000, 4, B10000000, //| B10000000, B10000000, B10000000, B10000000, B10000000, B10000000, 2, B10000000, //} B01000000, B01000000, B00100000, B01000000, B01000000, B10000000, 4, B00000000, //~ B00000000, B00000000, B01101000, B10010000, B00000000, B00000000, 6, B01100000, // (Char 0x7F) B10010000, B10010000, B01100000, B00000000, B00000000, B00000000, 5 }; void scrollFont() { for (int counter=0x20;counter<0x80;counter++){ loadBufferLong(counter); delay(500); } } // Scroll Message void scrollMessage(prog_uchar * messageString) { int counter = 0; int myChar=0; do { // read back a char myChar = pgm_read_byte_near(messageString + counter); if (myChar != 0){ loadBufferLong(myChar); } counter++; } while (myChar != 0); } // Load character into scroll buffer void loadBufferLong(int ascii){ if (ascii >= 0x20 && ascii <=0x7f){ for (int a=0;a<7;a++){ // Loop 7 times for a 5x7 font unsigned long c = pgm_read_byte_near(font5x7 + ((ascii - 0x20) * 8) + a); // Index into character table to get row data unsigned long x = bufferLong [a*2]; // Load current scroll buffer x = x | c; // OR the new character onto end of current bufferLong [a*2] = x; // Store in buffer } byte count = pgm_read_byte_near(font5x7 +((ascii - 0x20) * 8) + 7); // Index into character table for kerning data for (byte x=0; x<count;x++){ rotateBufferLong(); printBufferLong(); delay(scrollDelay); } } } // Rotate the buffer void rotateBufferLong(){ for (int a=0;a<7;a++){ // Loop 7 times for a 5x7 font unsigned long x = bufferLong [a*2]; // Get low buffer entry byte b = bitRead(x,31); // Copy high order bit that gets lost in rotation x = x<<1; // Rotate left one bit bufferLong [a*2] = x; // Store new low buffer x = bufferLong [a*2+1]; // Get high buffer entry x = x<<1; // Rotate left one bit bitWrite(x,0,b); // Store saved bit bufferLong [a*2+1] = x; // Store new high buffer } } // Display Buffer on LED matrix void printBufferLong(){ for (int a=0;a<7;a++){ // Loop 7 times for a 5x7 font unsigned long x = bufferLong [a*2+1]; // Get high buffer entry byte y = x; // Mask off first character lc.setRow(3,a,y); // Send row to relevent MAX7219 chip x = bufferLong [a*2]; // Get low buffer entry y = (x>>24); // Mask off second character lc.setRow(2,a,y); // Send row to relevent MAX7219 chip y = (x>>16); // Mask off third character lc.setRow(1,a,y); // Send row to relevent MAX7219 chip y = (x>>8); // Mask off forth character lc.setRow(0,a,y); // Send row to relevent MAX7219 chip } }
Demikian tutorial cara pemrograman modul MAX7219 Dot Matrix 4 In 1.
++++++++ Semoga Bermanfaat ++++++++
Leave a Reply