
MPU-6050
MPU-6050 adalah sebuah chip / IC yang diproduksi oleh Invensense yang mengkombinasikan 3 axis accelerometer dan 3 axis gyroscope dengan sebuah on-board digital motion processor. Pada Module ini juga sudah terdapat sensor suhu yang embedded. Module ini sangat akurat dan sudah terdapat hardware ADC untuk masing-masing channel untuk membaca x,y,z channels pada waktu yang sama. Untuk interface nya menggunakan protokol I2C bus sehingga parktis untuk digunakan.
3-axis Gyroscope
MPU-6050 terdiri dari 3 axis gyroscope yang dapat mendeteksi kecepatan putaran pada x,y,z axis dengan menggunakan teknologi MEMS.
- Ketika sensor diputar pada sembarang sumbu / axis, sebuah getaran / vibrasi dihasilkan dan dideteksi oleh MEMS.
- 16-bit ADC digunakan untuk mensampling tegangan pada masing-masing sumbu / axis.
- Mempunyai full scale range output : +/- 250, +/- 500, +/- 1000, +/- 2000.
- Kecepatan sudutnya diukur pada masing-masing sumbu / axis dalam satuan ukuran : degree per second.
3-axis Accelerometer
MPU-6050 terdiri dari 3 axis accelerometer yang dapat mendeteksi sudut pada sumbu x,y,z dengan teknologi Micro Electro Mechanical System (MEMS).
- Output Tegangan proporsional terhadap acceleration.
- Menggunakan 16-bit ADC untuk sampling nilai output.
- Mempunyai full scale range of output : +/- 2g, +/- 4g, +/- 8g, +/- 16g.
- Pada posisi normal yaitu ketika alat diletakkan pada permukaan datar / rata, nilai output : 0g pada x axis, 0g pada y axis and +1 pada z axis.
On Chip Temperature Sensor
Output dari sensor suhu didigitalisasi dengan ADC dapat dibaca pada data register dari sensor.
Digital motion processor (DMP)
The embedded digital motion processor is used to do computations on the sensor data. The values can be read off the dmp registers.
The raw values can be directly read of the accelerometer and gyroscope registers by the arduino. The MPU-6050 chip also includes a 1024 byte FIFO buffer where data can be placed and read off. The MPU-6050 always acts as a slave when connected to the Arduino with SDA and SCL pins connected to the I2C bus. But it also has its own auxiliary I2C controller which enables it to act as master to another device like a magnetometer.
The on-board DMP can be programmed with firmware to do complex calculations with the sensor values.
The AD0 selects between I2C addresses 0x68 and 0x69 enabling 2 of these sensors to be connected in the same project. Most breakout boards either have a pull down or pull up resistor to keep default at high or low.
GY-521 Module Board
Pinout

- VCC (Module GY-521 mempunyai onboard voltage regulator yang dapat dikoneksikan dengan 5v or 3.3v)
- GND (Ground pin)
- SCL (Serial clock line for I2C)
- SDA (Serial Data Line for I2C)
- XDA (Auxiliary data)
- XCL (Auxiliary clock)
- AD0 (Ketika pin ini di set low, I2C address = 0x68, ketika di set high, I2C address = 0x69)
- INT (Interrupt digital output)
Connections
Schematic

Wiring Diagram

Koneksi / Wiring nya adalah sebagai berikut :
- VCC – 5v pin dari Arduino Uno.
- GND – ground pin dari Arduino Uno.
- SDA – A4 pin dari Arduino Uno.
- SCL – A5 pin dari Arduino Uno.
Program
#include <Wire.h> //library allows communication with I2C / TWI devices #include <math.h> //library includes mathematical functions const int MPU=0x68; //I2C address of the MPU-6050 int16_t AcX,AcY,AcZ,Tmp,GyX,GyY,GyZ; //16-bit integers int AcXcal,AcYcal,AcZcal,GyXcal,GyYcal,GyZcal,tcal; //calibration variables double t,tx,tf,pitch,roll; void setup() { Wire.begin(); //initiate wire library and I2C Wire.beginTransmission(MPU); //begin transmission to I2C slave device Wire.write(0x6B); // PWR_MGMT_1 register Wire.write(0); // set to zero (wakes up the MPU-6050) Wire.endTransmission(true); //ends transmission to I2C slave device Serial.begin(9600); //serial communication at 9600 bauds } void loop() { Wire.beginTransmission(MPU); //begin transmission to I2C slave device Wire.write(0x3B); // starting with register 0x3B (ACCEL_XOUT_H) Wire.endTransmission(false); //restarts transmission to I2C slave device Wire.requestFrom(MPU,14,true); //request 14 registers in total //Acceleration data correction AcXcal = -950; AcYcal = -300; AcZcal = 0; //Temperature correction tcal = -1600; //Gyro correction GyXcal = 480; GyYcal = 170; GyZcal = 210; //read accelerometer data AcX=Wire.read()<<8|Wire.read(); // 0x3B (ACCEL_XOUT_H) 0x3C (ACCEL_XOUT_L) AcY=Wire.read()<<8|Wire.read(); // 0x3D (ACCEL_YOUT_H) 0x3E (ACCEL_YOUT_L) AcZ=Wire.read()<<8|Wire.read(); // 0x3F (ACCEL_ZOUT_H) 0x40 (ACCEL_ZOUT_L) //read temperature data Tmp=Wire.read()<<8|Wire.read(); // 0x41 (TEMP_OUT_H) 0x42 (TEMP_OUT_L) //read gyroscope data GyX=Wire.read()<<8|Wire.read(); // 0x43 (GYRO_XOUT_H) 0x44 (GYRO_XOUT_L) GyY=Wire.read()<<8|Wire.read(); // 0x45 (GYRO_YOUT_H) 0x46 (GYRO_YOUT_L) GyZ=Wire.read()<<8|Wire.read(); // 0x47 (GYRO_ZOUT_H) 0x48 (GYRO_ZOUT_L) //temperature calculation tx = Tmp + tcal; t = tx/340 + 36.53; //equation for temperature in degrees C from datasheet tf = (t * 9/5) + 32; //fahrenheit //get pitch/roll getAngle(AcX,AcY,AcZ); //printing values to serial port Serial.print("Angle: "); Serial.print("Pitch = "); Serial.print(pitch); Serial.print(" Roll = "); Serial.println(roll); Serial.print("Accelerometer: "); Serial.print("X = "); Serial.print(AcX + AcXcal); Serial.print(" Y = "); Serial.print(AcY + AcYcal); Serial.print(" Z = "); Serial.println(AcZ + AcZcal); Serial.print("Temperature in celsius = "); Serial.print(t); Serial.print(" fahrenheit = "); Serial.println(tf); Serial.print("Gyroscope: "); Serial.print("X = "); Serial.print(GyX + GyXcal); Serial.print(" Y = "); Serial.print(GyY + GyYcal); Serial.print(" Z = "); Serial.println(GyZ + GyZcal); delay(1000); } //function to convert accelerometer values into pitch and roll void getAngle(int Ax,int Ay,int Az) { double x = Ax; double y = Ay; double z = Az; pitch = atan(x/sqrt((y*y) + (z*z))); //pitch calculation roll = atan(y/sqrt((x*x) + (z*z))); //roll calculation //converting radians into degrees pitch = pitch * (180.0/3.14); roll = roll * (180.0/3.14) ; }
Output
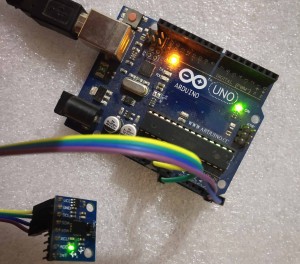
Output dapat dibaca pada serial port dari Arduino dengan menggunakan serial monitor.

Hi, thanks for coming to my website.